Guide
Authentication
The Unity API implements the OAuth 2.0 protocol for user authentication and authorization. In OAuth 2.0 a client requests an access token on behalf of a user. The access token is used for granting access to Unity API operations. See the API Reference for a complete list of the available Unity API operations.
Access and Refresh Tokens
There are two types of token:
- Access Token: grants access to protected Unity API calls.
- Refresh Token: can be used to obtain a new access token when the current access token is invalid or has expired.
Access Token Requirements
In order for a client to obtain an access token, the following information is required:
- Username: the email address of the user
- Password: the password of the user
- Client id: the unique id assigned to the client application
- Client secret: the password for authorizing the client application
Token Lifetimes
Access and refresh tokens both have a limited lifetime. The current values are as follows:
- Access token: 5 minutes
- Refresh token: 14 days
Authentication Flow
Step 1: Get Initial Access Token
When authenticating a user for the first time, all access token information (as listed in the "Access Token Requirements" section above) will need to be provided as part of the request. In addition, the set of operations an access token is permitted to request needs to be defined via the scope field (see Service Extensions and Scopes). Submit the access token information using the following request:
Password Flow
The following diagram shows the password authentication flow between a client and the Unity API server.
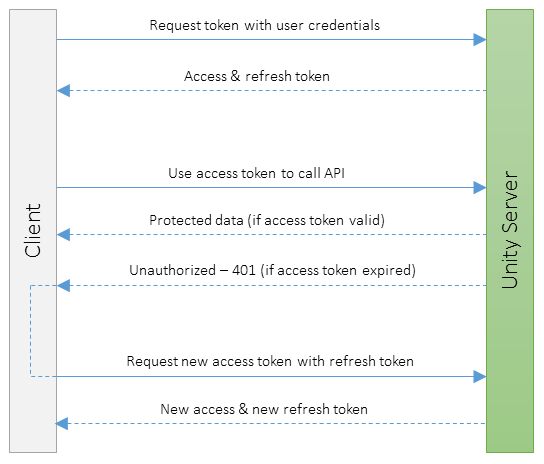
Method | API | Description |
---|---|---|
POST
|
Uses user credentials to obtain an access token
|
Authorization Code Flow
Note
At present only OpenText Secure Cloud IDP and Webroot IDP are supported. Additional IDPs will be supported in future releases.The following diagram shows the authorization code authentication flow between a client and the Unity API server.
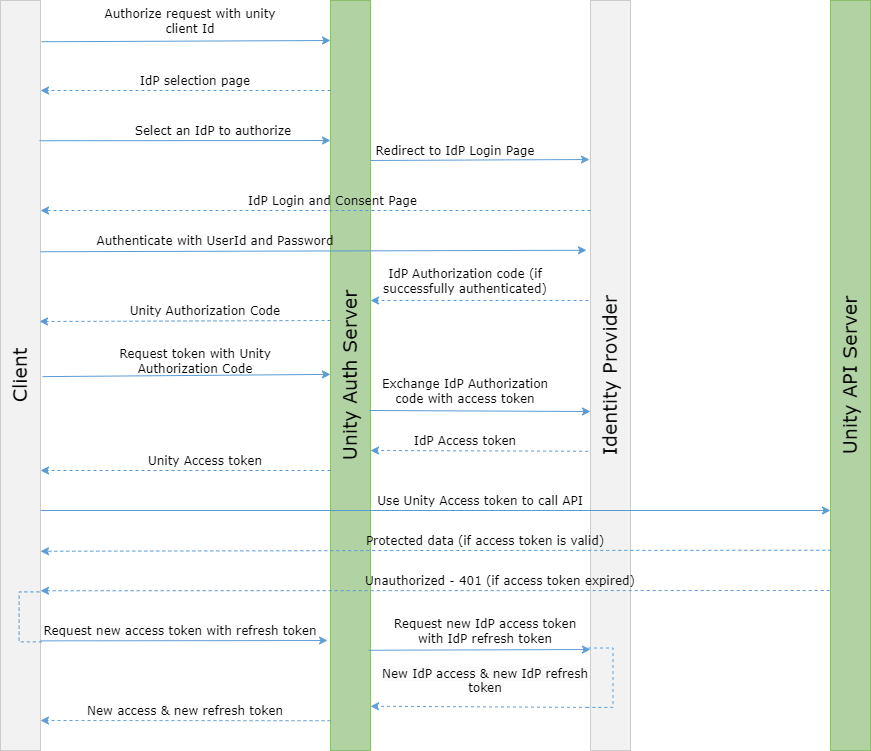
Method | API | Description |
---|---|---|
GET
|
Used to initiate the authorization process and obtain authorization code.
|
|
POST
|
Uses client credentials to exchange the authorization code for an access token.
|
Token Exchange Flow
Note
At present only OpenText Secure Cloud IDP and Webroot IDP are supported. Additional IDPs will be supported in future releases.The following diagram shows the token exchange authentication flow between a client and the Unity API server.
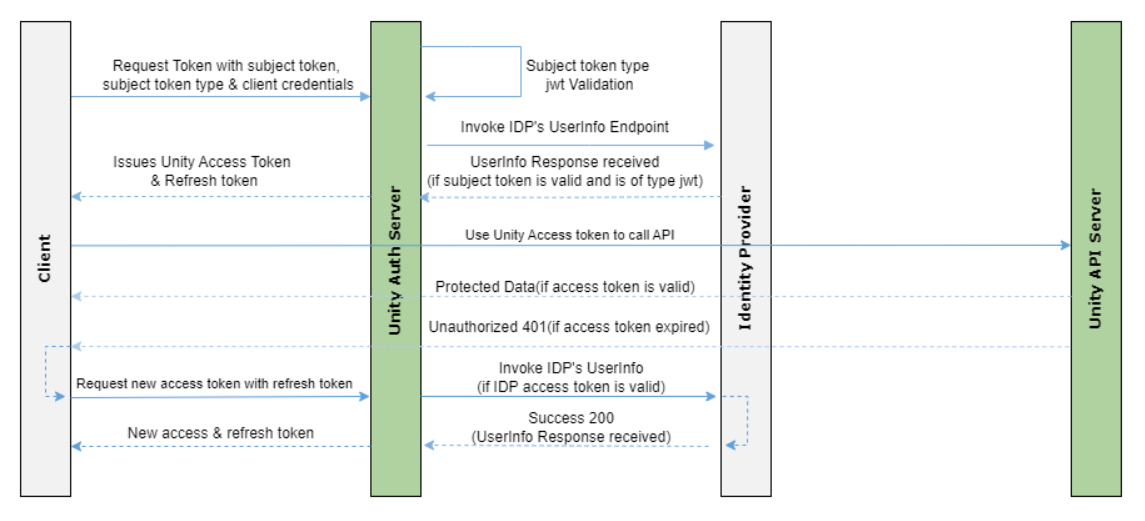
Method | API | Description |
---|---|---|
POST
|
Uses client credentials and IDP's access token to obtain a new Unity access and refresh token.
|
|
POST
|
Uses client credentials and a refresh token to obtain a new access and refresh token.
|
The response of a successful request contains an access token and a matching refresh token. The access token is identified by the "access_token" field and the refresh token by the "refresh_token" field.
Step 2: Send an authorized request to the Unity API
After an access token has been obtained, make authorized requests to the Unity API by adding an "Authorization" header to each request as follows:
Authorization: Bearer {access token}
If the submitted access token has expired, the server responds with an HTTP 401 (Unauthorized) status code and the message "error": "invalid_token". In that case a new access token needs to be obtained as described in "Step 3".
If the submitted access token does not contain the required scope (see Service Extensions and Scopes) for the requested API call, the server responds with an HTTP 403 (Forbidden) status code and the message "error": "insufficient_scope". In that case one of the following steps needs to be performed:
- If the user has appropriate access rights, a new access token needs to be obtained containing the required scope as described in "Step 3".
- If the user does not have appropriate access rights, please request additional scopes by contacting your trusted Webroot representative.
Step 3: Refresh Access Token
A new access token can be requested if the current access token is invalid or has expired. The recommended approach is to use the refresh token returned in the response of "Step 1" as it does not require any user credentials. In addition, the scope field needs to be set as part of the request (see Service Extensions and Scopes). It can be set equally as it was in "Step 1", but it can also be set differently permitting less operations for the access token to request. Submit the refresh token using the following request:
Method | API | Description |
---|---|---|
POST
|
Uses a refresh token to obtain an access token
|
The response of a successful request contains a new access token and matching refresh token.
If the submitted refresh token has expired or has become invalid, the server responds with an HTTP 400 (Bad Request) status code and the message "error": "invalid_grant" in the response body. In that case a new access and refresh token pair must be requested as described in Step 1 above.
Client Implementation Considerations
The use of refresh tokens eliminates the need for a client to store users' credentials (usernames and passwords).
Access and refresh tokens must be kept private. Unauthorized access to them can compromise user accounts.
For security reasons, the Unity API server implements refresh token rotation. This means that when a new refresh token is issued (for example, as part of "Step 3" above), the previous refresh token is invalidated.
If a refresh token is lost, invalid or has expired, then the inital request (see "Step 1" above) will need to be repeated.
A client can implement the handling of access token expiration in two ways:
- A client can call the Unity API with an access token until the server responds with an authentication error. This indicates that the access token has expired and that a refresh is required. The client can then refresh the token.
- A client can track the 'expires_in' parameter returned in Unity API responses and refresh the access token shortly before it expires.
Access to Console GSM API
Only users who are valid Global Site Manager (GSM) console users (see the Global Site Manager datasheet to learn more about GSM) have the required access permissions for accessing Console GSM API methods.
By default, every GSM console user has permission to access API methods listed under the group Console GSM. The GSM console user needs to obtain an access token with the appropriate scope "Console.GSM" included, to make authorized requests against related API methods. If additional scopes are desired for a certain GSM console user, please contact your trusted Webroot representative.
In order to get access to the Console GSM API methods, a client needs to authenticate (i.e. obtain an access token) with given GSM user credentials by setting the username and password accordingly and specifying "Console.GSM" as the requested scope. If the GSM authentication request was successful, the response returns an access token with the scope "Console.GSM" included. A client can verify the scope of the issued token by checking the "scope" field in the response body. The issued access token can then be used for making requests to the Console GSM API methods.
The Console GSM API provides calls for the following operations:
- Listing endpoint sites and policies
- Creating and editing endpoint sites
- Listing users and changing permissions
- Suspending, resuming, and deactivating an endpoint site
- Listing, creating, editing, and deleting endpoint groups of a site
- Listing endpoints in a site or group
- Moving endpoints into or out of a group
- Assigning a policy to endpoints
- Listing agent commands
- Issuing agent commands to endpoints
- Re- or deactivating endpoints
Some of these operations require certain user permissions in the Global Site Manager console. For instance, if a user wants to create or edit a site, the user needs to have the "GSM Super Admin" account type assigned.
Important: If a user performs a password change via the GSM console, all issued tokens get automatically revoked and a new set of access and refresh tokens needs to be obtained with the new GSM user credentials provided.
Service Extensions and Scopes
A Service Extension is a plugin which provides an interface to a Webroot service. Each Service Extension defines the request path prefix(es) that are associated with the Service Extension. Each request path prefix is labelled as a "Scope".
Scopes are used during authentication to manage user access rights. A user may or may not have permission to make requests against a specific scope depending on the corresponding user account settings. When requesting an access token, it is recommended to only specify the minimum amount of scopes required, as there is a performance overhead associated with each scope.
Scope Wildcards
Scopes are defined using the pattern [extensionName].[domain]
Scopes support pattern matching. The * character is used as the wildcard character. The following matching patterns are supported:
Pattern | Description | Example |
---|---|---|
[extensionName].* | Gets all scopes that contain the specified extension name. | SkyStatus.* could match SkyStatus.GSM and SkyStatus.Site. |
*.[domain] | Gets all scopes that contain the specified domain. | *.GSM could match SkyStatus.GSM |
* | Gets all scopes. | * matches all scopes available to the user. |
Note
Wildcard support is not available for Identity Providers (IDPs). This applies to both the Authorization Code (AuthCode) flow and the Token Exchange flow.Available Scopes
ECom
Provides a set of API calls for creating and modifying licenses, as well as retrieving license and license order information.
Scope | Request Path Prefix |
---|---|
ECom.Shop | /service/api/ecom/shop |
SkyStatus
Provides a set of API calls for retrieving agent and license status information from specific endpoints.
Scope | Request Path Prefix |
---|---|
SkyStatus.GSM | /service/api/status/gsm |
SkyStatus.Site | /service/api/status/site |
SkyStatus.Reporting | /service/api/status/reporting |
Console
Provides a set of API calls for console site management.
Scope | Request Path Prefix |
---|---|
Console.Access | /service/api/console/access |
Console.GSM | /service/api/console/gsm |
Notifications
Provides a set of API calls for Notifications.
Scope | Request Path Prefix |
---|---|
Notifications.Subscriptions | /service/api/notifications/subscriptions |
SecureCloud
Provides a set of API methods for the OpenText Secure Cloud platform.
Scope | Request Path Prefix |
---|---|
SecureCloud.Platform | /service/api/securecloud/customers |
SecureCloud.Usage | /service/api/securecloud/usage/charges |
Response Formats
Success and Error Response Formats
Unity API responds to calls in standard data formats (mainly JSON or XML). The specific data items returned for each successful request are individual to each API method and can be looked up via the API Reference.
Depending on the 'Accept' headers of a request, information is either returned as JSON (which is the default) or as XML objects. If an error occurs when executing an API call, responses are in a standardized format (see below):
Error Response Information
Resource Description
Unity API returns error information in a standardized way throughout all API calls. Depending on the 'Accept' headers of a request, information is either returned as JSON (which is the default) or XML objects.
Name | Description | Type | Additional Information |
---|---|---|---|
statusCode |
The HTTP status code returned by the API server. |
number |
None. |
requestId |
A GUID uniquely identifying the request that generated the error. The request ID will help Webroot troubleshooting issues in case you have any problems. |
string |
None. |
error |
This value contains an error code in case of OAuth failures or is null in all other cases. |
string |
None. |
error_description |
Contains descriptive text explaining, why the error response was generated or null if no description is available. |
string |
None. |
AdditionalInformation |
Contains an array of objects providing additional error details. The content of the array is dependent on the type of error reported. |
array of objects |
None. |
Response Formats
{ "statusCode": 400, "requestId": "a0946d06-fad9-4b01-bc66-f685f04c7899", "error": null, "error_description": "Batch size must be between 1 and 1000", "AdditionalInformation": {} }
<z:anyType> <statusCode>400</statusCode> <requestId>a0946d06-fad9-4b01-bc66-f685f04c7899</requestId> <error></error> <error_description>Batch size must be between 1 and 1000</error_description> <AdditionalInformation></AdditionalInformation> </z:AnyType>
OpenText Secure Cloud Filter Operations
Filter Operations
OpenText Secure Cloud is using the query string's operators and expressions to construct filters. E.g. filter[name]=in:Mike,Michael, filter[id]=ir:(21,10].
Expression values
Description
Unity API for OpenText Secure Cloud supports certain expressions within the filter fields in the request body.
The filter syntax takes the generic form [filter name] [operator] [value].
Expressions will consist of values and operators as shown below.
Operation | Meaning | Example |
---|---|---|
eq |
key is equal to value. |
filter[name]=eq:Michael |
ne |
key is not equal to value. |
filter[name]=ne:Michael |
in |
key is in the comma-separated list of values. |
filter[name]=in:Mike,Michael |
ni |
key is not in the comma-separated list of values. |
filter[name]=ni:Chris,Christopher |
lt |
key is less than value. |
filter[years]=lt:15 |
le |
key is less than or equal to value. |
filter[years]=le:15 |
gt |
key is greater than value. |
filter[years]=gt:5 |
ge |
key is greater or equal to value. |
filter[years]=ge:5 |
like |
key matches the regular expression value. |
filter[name]=like:^[Mm]i(ke|chael)$ |
ir |
key is greater than or equal to start and less than or equal to end. |
filter[years]=ir:5,10 |
...or |
filter[years]=ir:[5, 10] | |
ir |
key is greater than start and less than end. |
filter[years]=ir:(5, 10) |
ir |
key is greater than or equal to start and less than end. |
filter[years]=ir:[5,10) |
ir |
key is greater than start and less than or equal to end. |
filter[years]=ir:(5,10] |
Status Codes
Common Response Status Codes
The Unity API adopts the standard HTTP status codes as defined in the HTTP specification. The status codes that apply to the Unity API have been listed below.
Success Status Codes
The 2xx status codes indicate that the client's request was successfully received, understood, and accepted.
Status Code | Description |
---|---|
200 OK | The request has succeeded. |
201 Created | The request has succeeded and a new resource has been created. |
204 No Content | The request has succeeded with no data being returned in the response body. |
Error Status Codes
The 4xx and 5xx status codes indicate that an error has occurred. As expected, 4xx status codes are used for client errors (authentication errors, validation errors, etc.), and 5xx status codes are used for server errors.
Status Code | Description |
---|---|
400 Bad Request | The request could not be understood by the server due to malformed syntax. The client SHOULD NOT repeat the request without modifications. |
401 Unauthorized | The client could not be authenticated. This may indicate that the token used for authentication is invalid or has expired. |
403 Forbidden | The server understood the request, but is refusing to fulfill it. Authorization will not help and the request SHOULD NOT be repeated. |
404 Not Found | The client request could not be routed or the requested resource does not exist. |
405 Method Not Allowed | The 405 status code in the Unity API is often used interchangeably with the 501 (Not Implemented) status code. |
409 Conflict | The request could not be completed due to a conflict with the current state of the resource. |
422 Unprocessable Entity | Validation errors have occurred. |
429 Too Many Requests | The request was rejected because the user has sent too many requests in a given amount of time ("rate limiting"). |
500 Internal Server Error | The server encountered an unexpected condition which prevented it from fulfilling the request. |
501 Not Implemented | The server does not support the functionality required to fulfill the request. |
503 Service Unavailable | The server is currently unable to handle the request due to a temporary overloading or maintenance of the server. |